Understanding static public int main(String[]
args)
package myapp;
public class Cmd {
public static void main(String[] args) {
for (int i = 0; i < args.length; i++) {
System.out.println("Parameter " + (i + 1) + ": " + args[i]);
}
}
}
java myapp.Cmd 21 334 -13 Parameter 1: 21 Parameter 2: 334 Parameter 3: -13

❶ |
Entry class |
❷ |
Parameters |
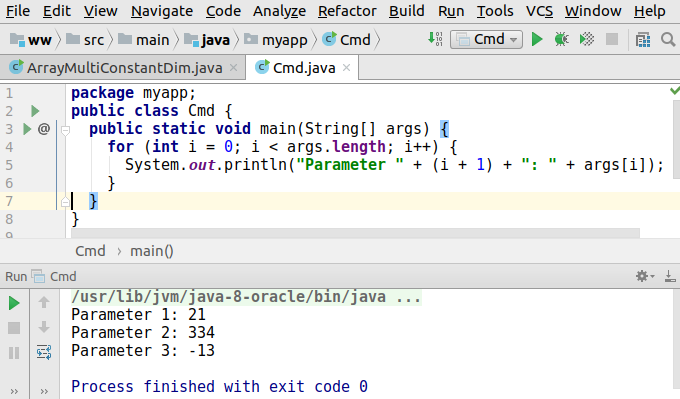
<artifactId>maven-shade-plugin</artifactId>
...
<transformer ...>
<manifestEntries>
<Main-Class>myapp.Cmd</Main-Class>
</manifestEntries>... |
unzip ww-1.0-SNAPSHOT.jar
cat tmp/META-INF/MANIFEST.MF
...
Created-By: Apache Maven 3.5.0
Build-Jdk: 1.8.0_151
Main-Class: myapp.Cmd |
mvn package ... java -jar target/ww-1.0-SNAPSHOT.jar 21 334 -13 Parameter 1: 21 Parameter 2: 334 Parameter 3: -13
No. 154
Reading console input
Q: |
Consider the following code example computing the sum of an arbitrary number of integer values:
This program:
The result might look like: How many values do you want to summ up? 4 Enter value #1 of 4: 11 Enter value #2 of 4: 22 Enter value #3 of 4: -33 Enter value #4 of 4: 1 Sum=1 Modify this program to output not just the desired sum but the complete arithmetic expression: How many values do you want to summ up? 4
Enter value #1 of 4: 1
...
1+2+3-5 = 1 Hints:
|
A: |
|
No. 155
Prettifying output representation
Q: |
The output representation of Reading console input shall be altered by the following rules:
Write your application in a unit testable fashion. Tip
|
A: |
|